Python | not supported between instances of ‘int’ and ‘str’ エラーの原因と解決策
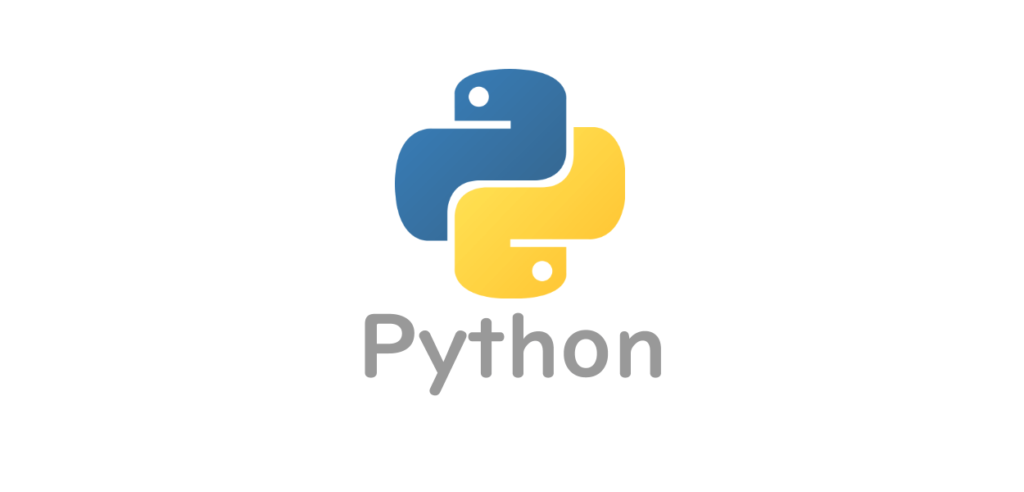
Pythonの実行時に発生するエラー「not supported between instances of ‘int’ and ‘str’」の原因と解決策について紹介しています。
TypeError: ‘<‘ not supported between instances of ‘int‘ and ‘str‘
TypeError: 「int」と「str」のインスタンス間で「<」はサポートされていません
「<」の部分には別の比較演算子が入るいくつかのパターンがあります。
下記はそのパターン例になります。
TypeError: ‘==‘ not supported between instances of ‘int’ and ‘str’
TypeError: ‘>‘ not supported between instances of ‘int’ and ‘str’
TypeError: ‘>=‘ not supported between instances of ‘int’ and ‘str’
TypeError: ‘<=‘ not supported between instances of ‘int’ and ‘str’
また、「’int‘ and ‘str‘」の部分にはそれぞれ処理に与えられている型が順番に表示されています。
このエラーは、様々な型を対象に発生します。下記はそのパターン例になります。
TypeError: ‘>‘ not supported between instances of ‘int‘ and ‘str‘
TypeError: ‘>‘ not supported between instances of ‘int‘ and ‘list‘
TypeError: ‘>‘ not supported between instances of ‘int‘ and ‘dict‘
Windows11 ローカル
Python python-3.11.1
not supported between instances of ‘int’ and ‘str’ エラーの原因
「not supported between instances of ‘int’ and ‘str’」というPythonの実行エラーは与えられた2つの値の型が実行しようとしている処理に問題を起こす場合に発生するエラーです。
例えば以下のような事がエラーの発生原因にあります。
- 「’int’ and ‘str’」の場合は整数値と文字列を比較している
- 「’int’ and ‘list’」の場合は整数値とリスト(配列)を比較している
- 「’int’ and ‘dict’」の場合は整数値と辞書(連想配列)を比較している
not supported between instances of ‘int’ and ‘str’ エラーの発生例と解決策
「not supported between instances of ‘int’ and ‘str’」エラーが発生するコード例とその解決策を紹介します。
数値と文字列を比較している
原因のひとつとして、文字列型の数値と整数値を比較している場合、このエラーは発生します。
a = 4
b = '8'
if a < b:
print('aはbより小さい')
# TypeError: '<' not supported between instances of 'int' and 'str'
上記のコードエラーを解決には、例えば、比較対象が文字列型の数値であれば、int()
関数で数値に変換して実行する方法などがあります。
a = 4
b = '8'
if a < int(b):
print('aはbより小さい')
# aはbより小さい
数値とリスト(配列)を比較している
数値とリスト(配列)そのものを比較している場合にもこのエラーは発生します。
以下のように、数値との比較対象にリストをそのまま指定していると発生します。
a = 4
data = (5, 6, 8, 3)
if a < data:
print('aはbより小さい')
# TypeError: '<' not supported between instances of 'int' and 'str'
このコードによるエラーは、リスト内にある数値データを正確に提示する事で解決できる場合があります。
この発生パターンはfor
文などでリストや辞書を繰り返す処理中の記述ミスで起こる可能性があります。
a = 4
data = (5, 6, 8, 3)
if a < data[2]:
print('aより小さい')
# aより小さい