正規表現 | 言語別テスト用サンプルコード集
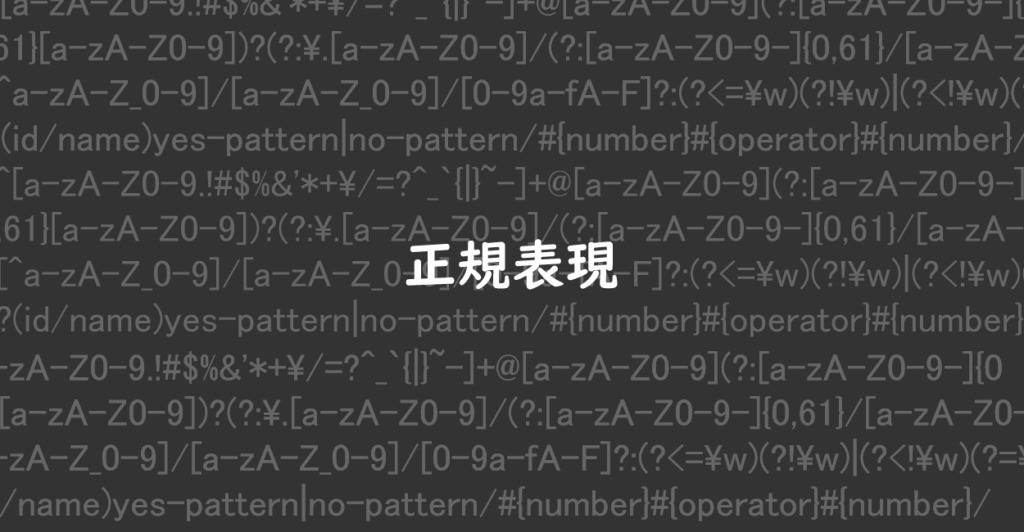
言語別に簡単な正規表現のテスト用サンプルコードを紹介しています。
PHP
PHPで正規表現の簡単なテストをする時用のサンプルコードです。
// 正規表現パターン
$pattern = '/Hallo/';
// テストする文字列
$string = 'Hallo World';
// パターンマッチングのテスト
if (preg_match($pattern, $string)) {
echo '<p>True</p>';
} else {
echo '<p>False</p>';
}
// マッチした結果をすべて取得
preg_match_all($pattern, $string, $matches);
print_r($matches);
Python
Pythonで正規表現の簡単なテストをする時用のサンプルコードです。
import re
# 正規表現パターン
pattern = r'Hallo'
# テストする文字列
string = 'Hallo World'
# パターンマッチングのテスト
if re.search(pattern, string):
print('<p>True</p>')
else:
print('<p>False</p>')
# マッチした結果をすべて取得
matches = re.findall(pattern, string)
print(matches)
JavaScript
JavaScriptで正規表現の簡単なテストをする時用のサンプルコードです。
// 正規表現パターン
const pattern = /Hallo/;
// テストする文字列
const string = 'Hallo World';
// パターンマッチングのテスト
if (pattern.test(string)) {
console.log('<p>True</p>');
} else {
console.log('<p>False</p>');
}
// マッチした結果をすべて取得
const matches = string.match(pattern);
console.log(matches);
TypeScript
TypeScriptで正規表現の簡単なテストをする時用のサンプルコードです。
const pattern: RegExp = /Hallo/;
// テストする文字列
const string: string = 'Hallo World';
// パターンマッチングのテスト
if (pattern.test(string)) {
console.log('<p>True</p>');
} else {
console.log('<p>False</p>');
}
// マッチした結果をすべて取得
const matches: RegExpMatchArray | null = string.match(pattern);
if (matches) {
console.log(matches.join('\n'));
}
C#
C#で正規表現の簡単なテストをする時用のサンプルコードです。
using System;
using System.Text.RegularExpressions;
class Program
{
static void Main()
{
// 正規表現パターン
string pattern = @"Hallo";
// テストする文字列
string input = "Hallo World";
// パターンマッチングのテスト
if (Regex.IsMatch(input, pattern))
{
Console.WriteLine("<p>True</p>");
}
else
{
Console.WriteLine("<p>False</p>");
}
// マッチした結果をすべて取得
MatchCollection matches = Regex.Matches(input, pattern);
foreach (Match match in matches)
{
Console.WriteLine(match.Value);
}
}
}
C++
C++で正規表現の簡単なテストをする時用のサンプルコードです。
#include <iostream>
#include <regex>
int main() {
// 正規表現パターン
std::regex pattern("Hallo");
// テストする文字列
std::string str = "Hallo World";
// パターンマッチングのテスト
if (std::regex_search(str, pattern)) {
std::cout << "<p>True</p>" << std::endl;
} else {
std::cout << "<p>False</p>" << std::endl;
}
// マッチした結果をすべて取得
std::smatch matches;
while (std::regex_search(str, matches, pattern)) {
for (const auto& match : matches) {
std::cout << match << std::endl;
}
str = matches.suffix().str();
}
return 0;
}
Perl
Perlで正規表現の簡単なテストをする時用のサンプルコードです。
# 正規表現パターン
my $pattern = qr/Hallo/;
# テストする文字列
my $string = 'Hallo World';
# パターンマッチングのテスト
if ($string =~ $pattern) {
print "<p>True</p>\n";
} else {
print "<p>False</p>\n";
}
# マッチした結果をすべて取得
my @matches = $string =~ /$pattern/g;
print join("\n", @matches), "\n";
Java
Javaで正規表現の簡単なテストをする時用のサンプルコードです。
import java.util.regex.Matcher;
import java.util.regex.Pattern;
public class Main {
public static void main(String[] args) {
// 正規表現パターン
String pattern = "Hallo";
// テストする文字列
String input = "Hallo World";
// パターンマッチングのテスト
if (Pattern.compile(pattern).matcher(input).find()) {
System.out.println("<p>True</p>");
} else {
System.out.println("<p>False</p>");
}
// マッチした結果をすべて取得
Matcher matcher = Pattern.compile(pattern).matcher(input);
while (matcher.find()) {
System.out.println(matcher.group());
}
}
}
Ruby
Rubyで正規表現の簡単なテストをする時用のサンプルコードです。
# 正規表現パターン
pattern = /Hallo/
# テストする文字列
input = 'Hallo World'
# パターンマッチングのテスト
if input.match?(pattern)
puts '<p>True</p>'
else
puts '<p>False</p>'
end
# マッチした結果をすべて取得
matches = input.scan(pattern)
matches.each do |match|
puts match
end