JavaScript | Canvasでテキストの縦横中央揃えと各ポジションの指定パターン
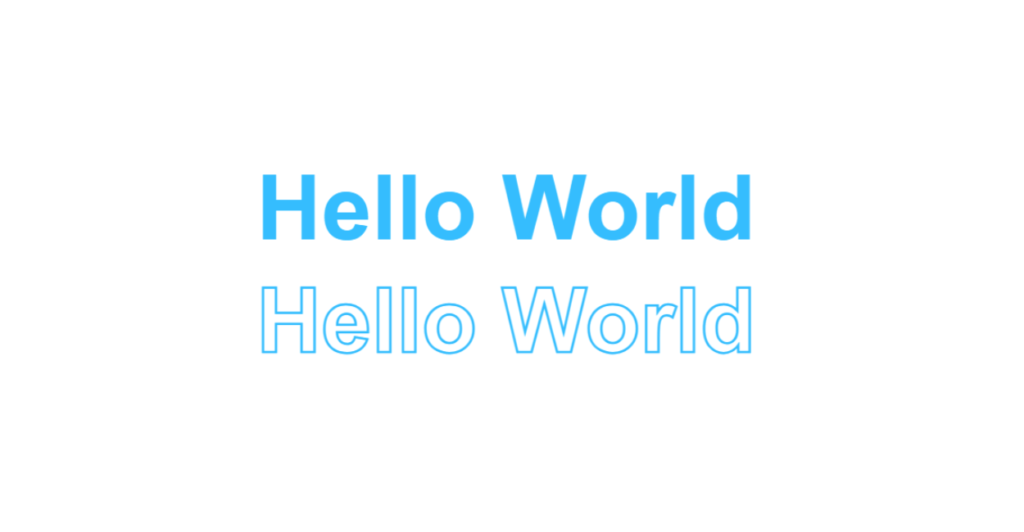
JavaScriptでCanvas描画するテキストの縦横中央揃えほか、左上、左下、右上、右下などの各ポジションの指定方法を紹介しています。
テキストの各ポジションの指定パターン
JavaScriptでCanvasに各タイプ別のテキストを描画するサンプルコードになります。
左から枠線なし、枠線ありのテキストとなっています。
See the Pen JavaScript Canvas draw text positions by yochans (@yochans) on CodePen.
HTMLはcanvas要素にid=canvasを指定しています。
<canvas id="canvas"></canvas>
サンプルでは左上から順にそれぞれの位置にテキストを描画を指定してます。
位置を調節する場合は、CSSとは違ってCanvasのテキストの基準点は左下である事に注意して下さい。
※例えば「x=0 y=0」を指定した場合、左寄りになりますがキャンバスの上に描画される為見えません
一度に複数の図形を描画する場合、パスをbeginPath()でリセットする必要があります(新しいパスを開始)。
let canvas = document.querySelector('#canvas');
let context = canvas.getContext('2d');
//canvas size and background color
canvas.width = 500;
canvas.height = 200;
context.beginPath();
context.fillStyle = 'rgb( 0, 0, 0)';
context.fillRect(0, 0, canvas.width, canvas.height);
/text option
let text = 'Hello World';
let font_size = 26;
let x = 0;
let y = 0;
//top left
context.beginPath();
context.fillStyle = 'deepskyblue';
context.font = `bold ${font_size}px Arial`;
x = 0;
y = font_size;
context.fillText(text, x, y);
//top center
context.beginPath();
context.fillStyle = 'deepskyblue';
context.font = `bold ${font_size}px Arial`;
x = (canvas.width - context.measureText( text ).width) / 2;
y = font_size;
context.fillText(text, x, y);
//top right
context.beginPath();
context.fillStyle = 'deepskyblue';
context.font = `bold ${font_size}px Arial`;
x = (canvas.width - context.measureText( text ).width);
y = font_size;
context.fillText(text, x, y);
//middle left
context.beginPath();
context.fillStyle = 'deepskyblue';
context.font = `bold ${font_size}px Arial`;
x = 0;
y = (canvas.height + font_size) / 2;
context.fillText(text, x, y);
//middle center
context.beginPath();
context.fillStyle = 'deepskyblue';
context.font = `bold ${font_size}px Arial`;
x = (canvas.width - context.measureText( text ).width) / 2;
y = (canvas.height + font_size) / 2;
context.fillText(text, x, y);
//middle right
context.beginPath();
context.fillStyle = 'deepskyblue';
context.font = `bold ${font_size}px Arial`;
x = (canvas.width - context.measureText( text ).width);
y = (canvas.height + font_size) / 2;
context.fillText(text, x, y);
//bottom left
context.beginPath();
context.fillStyle = 'deepskyblue';
context.font = `bold ${font_size}px Arial`;
x = 0;
y = canvas.height;
context.fillText(text, x, y);
//bottom center
context.beginPath();
context.fillStyle = 'deepskyblue';
context.font = `bold ${font_size}px Arial`;
x = (canvas.width - context.measureText( text ).width) / 2;
y = canvas.height;
context.fillText(text, x, y);
//bottom right
context.beginPath();
context.fillStyle = 'deepskyblue';
context.font = `bold ${font_size}px Arial`;
x = (canvas.width - context.measureText( text ).width);
y = canvas.height;
context.fillText(text, x, y);