JavaScript | createElementNSでSVGの図形を描画する基本
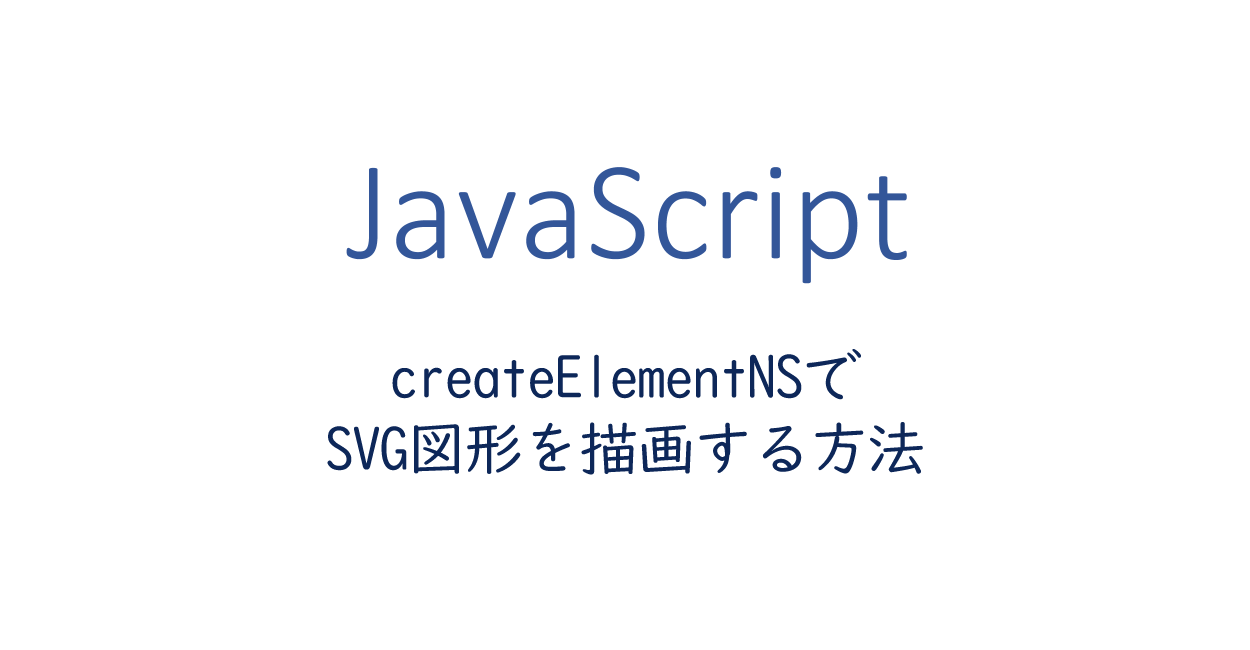
JavaScripでcreateElementNSを使ってシンプルなSVGの図形を描画する基本的な記述方法です。
図形描画のベースとなる直線、折れ線、曲線、円、楕円、四角形を紹介しています。
基本
createElementNSでSVGを扱う基本構造です。
//svg
const svg = document.createElementNS('http://www.w3.org/2000/svg', 'svg');
svg.setAttribute("width", "200");
svg.setAttribute("height", "200");
svg.setAttribute("viewbox", "0 0 200 200");
svg.setAttribute('style', 'background-color:#faebd7;');
//line
const line = document.createElementNS('http://www.w3.org/2000/svg', 'line');
line.setAttribute('x1',20);
line.setAttribute('y1',20);
line.setAttribute('x2',180);
line.setAttribute('y2',180);
line.setAttribute('stroke', '#008080');
line.setAttribute('stroke-width', 5);
line.setAttribute('stroke-dasharray', "none");//破線 10,10 etc
line.setAttribute('stroke-linejoin', 'miter'); //角 miter round bevel inherit
line.setAttribute('stroke-linecap', 'butt'); //切れ目 butt round square inherit
line.setAttribute("opacity", 1);
line.setAttribute('fill-opacity', 1);
line.setAttribute('stroke-opacity', 1);
line.setAttribute('transform', "rotate(0)");
//作成した図形をsvgに追加
svg.appendChild( line );
//特定の要素内にsvgを追加(bodyでも可)
document.querySelector('#container').appendChild( svg );
svgで作成した図形、テキストを描画するにはキャンバス・ベースになる<svg>タグが必要です。
直線(line)
See the Pen JavaScript | createElementNS svg line by yochans (@yochans) on CodePen.
「line」は直線を描く事が可能です。
始点「x1」「y1」と終点「x2」「y2」の指定が必須です。
//line
const line = document.createElementNS('http://www.w3.org/2000/svg', 'line');
line.setAttribute('x1',20);
line.setAttribute('y1',20);
line.setAttribute('x2',180);
line.setAttribute('y2',180);
line.setAttribute('stroke', '#008080');
line.setAttribute('stroke-width', 5);
line.setAttribute('stroke-dasharray', "none");//破線 10,10 etc
line.setAttribute('stroke-linejoin', 'miter'); //角 miter round bevel inherit
line.setAttribute('stroke-linecap', 'butt'); //切れ目 butt round square inherit
line.setAttribute("opacity", 1);
line.setAttribute('fill-opacity', 1);
line.setAttribute('stroke-opacity', 1);
line.setAttribute('transform', "rotate(0)");
四角形(rect)
「rect」は始点から指定サイズで作られる四角形を描く事が可能です。
始点「x」「y」とサイズ「width」「height」の指定が必須です。
See the Pen JavaScript | createElementNS svg Rect by yochans (@yochans) on CodePen.
//rect
const rect = document.createElementNS('http://www.w3.org/2000/svg', 'rect');
rect.setAttribute( 'x', 20);
rect.setAttribute( 'y', 20);
rect.setAttribute('width', 170);
rect.setAttribute('height', 170);
rect.setAttribute('fill', 'none');
rect.setAttribute('stroke', '#0000ff');
rect.setAttribute('stroke-width', 5);
rect.setAttribute('stroke-dasharray', "none");//破線 10,10 etc
rect.setAttribute('stroke-linejoin', 'miter'); //角 miter round bevel inherit
rect.setAttribute('stroke-linecap', 'butt'); //切れ目 butt round square inherit
rect.setAttribute('opacity', 1);
rect.setAttribute('fill-opacity', 1);
rect.setAttribute('stroke-opacity', 1);
rect.setAttribute('transform', "rotate(0)");
円(circle)
「circle」は半径を指定した正円を描くことが可能です。
「cx」「cy」と半径にあたる「r」の指定が必須です。
See the Pen JavaScript | createElementNS svg Circle by yochans (@yochans) on CodePen.
//circle
const circle = document.createElementNS('http://www.w3.org/2000/svg', 'circle');
circle.setAttribute('cx', 100);
circle.setAttribute('cy', 100);
circle.setAttribute('r', 80);
circle.setAttribute('fill', 'none');
circle.setAttribute('stroke', '#b22222');
circle.setAttribute('stroke-width', 5);
circle.setAttribute('stroke-dasharray', "none");//破線 10,10 etc
circle.setAttribute('opacity', 1);
circle.setAttribute('fill-opacity', 1);
circle.setAttribute('stroke-opacity', 1);
circle.setAttribute('transform', "rotate(0)");
楕円(ellipse)
「ellipse」は縦軸、横軸別々の半径を指定可能で楕円を描くことができます。
「cx」「cy」と半径にあたる「rx」「ry」の指定が必須です。
See the Pen JavaScript | createElementNS svg Ellipse by yochans (@yochans) on CodePen.
//ellipse
const ellipse = document.createElementNS('http://www.w3.org/2000/svg', 'ellipse');
ellipse.setAttribute('cx', 150);
ellipse.setAttribute('cy', 0);
ellipse.setAttribute('rx', 80);
ellipse.setAttribute('ry', 50);
ellipse.setAttribute('fill', 'none');
ellipse.setAttribute('stroke', '#008080');
ellipse.setAttribute('stroke-width', 5);
ellipse.setAttribute('stroke-dasharray', "none");//破線 10,10 etc
ellipse.setAttribute('opacity', 1);
ellipse.setAttribute('fill-opacity', 1);
ellipse.setAttribute('stroke-opacity', 1);
ellipse.setAttribute('transform', "rotate(45)");
折れ線(path)
「path」で折れ線を描いたサンプルです。
連続したパスを指定する「d」は必須になっています。
See the Pen JavaScript | createElementNS svg Ellipse by yochans (@yochans) on CodePen.
//line path
line_path = document.createElementNS('http://www.w3.org/2000/svg', 'path');
line_path.setAttribute('d', 'M 20,20 70,180 120,20 170,180');
line_path.setAttribute('fill', 'none');
line_path.setAttribute('stroke', '#8a2be2');
line_path.setAttribute('stroke-width', 5);
line_path.setAttribute('stroke-dasharray', "none");//破線 10,10 etc
line_path.setAttribute('stroke-linejoin', 'round'); //角 miter round bevel inherit
line_path.setAttribute('stroke-linecap', 'round'); //切れ目 butt round square inherit
line_path.setAttribute('opacity', 1);
line_path.setAttribute('fill-opacity', 1);
line_path.setAttribute('stroke-opacity', 1);
line_path.setAttribute('transform', "rotate(0)");
曲線(path)
「path」でペジェ曲線を描いたサンプルです。
「path」でペジェ曲線を描く記述方法はいくつもありますが、こちらはそのひとつになります。
上記の折れ線と異なり、ペジェ曲線の場合は指定した座標の値まで線が伸びない事を意識して触れば理解しやすいです。
See the Pen JavaScript | createElementNS svg Curve : path by yochans (@yochans) on CodePen.
//curve path
curve_path = document.createElementNS('http://www.w3.org/2000/svg', 'path');
curve_path.setAttribute('d', 'M 20,20 C 20,200 180,200 180,20');
curve_path.setAttribute('fill', 'none');
curve_path.setAttribute('stroke', '#ff8c00');
curve_path.setAttribute('stroke-width', 5);
curve_path.setAttribute('stroke-dasharray', "none");//破線 10,10 etc
curve_path.setAttribute('stroke-linejoin', 'round'); //角 miter round bevel inherit
curve_path.setAttribute('stroke-linecap', 'round'); //切れ目 butt round square inherit
curve_path.setAttribute('opacity', 1);
curve_path.setAttribute('fill-opacity', 1);
curve_path.setAttribute('stroke-opacity', 1);
curve_path.setAttribute('transform', "rotate(0)");
ディスカッション
コメント一覧
まだ、コメントがありません