JavaScript | カレンダーを動的に生成する
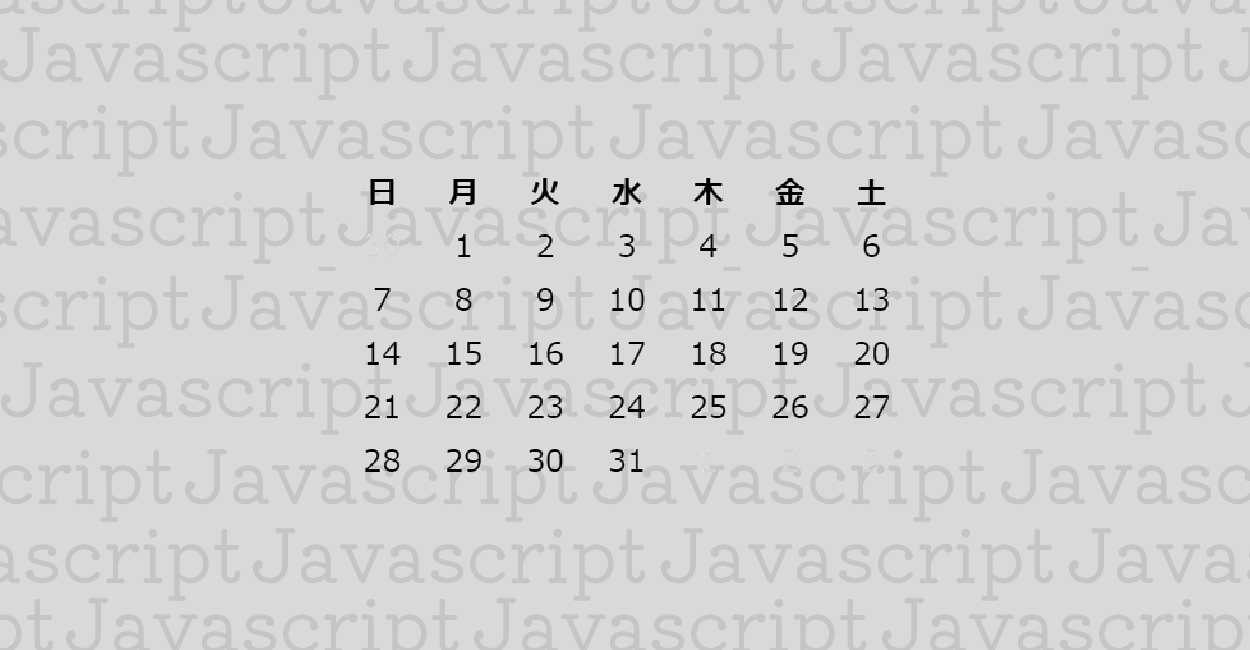
JavaScriptでカレンダーを動的に生成する関数とサンプルコードを紹介しています。
カレンダーを生成する
JavaScriptで作成するカレンダーの動作サンプルとサンプルコードになります。
少し手を加えれば表示月の移動なども可能となっています。
See the Pen CSS | Table caption position by yochans (@yochans) on CodePen.
<div id="calendar"></div>
JavaScriptでid「calendar」のdiv要素に対して、テーブルタグを使ったカレンダーを作成しています。
monthについて、プログラムは0を1月としますが、認識のために1を減算しています。(月の引数が1の場合、プログラム上の1月にあたる0にしています。)
function generateCalendar(year, month) {
month -= 1;
let calendarHTML = '';
// 月の最初の日の取得
const firstDay = new Date(year, month, 1);
// 月の最後の日の取得
const lastDay = new Date(year, month + 1, 0);
// 先頭に年と月を表示
calendarHTML += `<p>${year}年 ${month + 1}月</p>`;
// テーブルの開始タグ
calendarHTML += '<table>';
// 曜日の配列
const weekdays = ['日', '月', '火', '水', '木', '金', '土'];
// 曜日のヘッダー行
calendarHTML += '<tr>';
for (let i = 0; i < weekdays.length; i++) {
calendarHTML += `<th>${weekdays[i]}</th>`;
}
calendarHTML += '</tr>';
// カレンダーの日付を埋める
let dayCount = 1;
let rowCount = 0;
// 先月の最後の日の取得
const prevMonthLastDay = new Date(year, month, 0).getDate();
// 先月の日付を埋める
const firstDayOfWeek = firstDay.getDay(); // 先月の最初の曜日
for (let i = prevMonthLastDay - firstDayOfWeek + 1; i <= prevMonthLastDay; i++) {
calendarHTML += `<td class="prev-month">${i}</td>`;
rowCount++;
}
while (dayCount <= lastDay.getDate()) {
if (rowCount === 0) {
calendarHTML += '<tr>';
}
// 現在の月の日を表示
calendarHTML += `<td>${dayCount}</td>`;
dayCount++;
rowCount++;
if (rowCount === 7) {
calendarHTML += '</tr>';
rowCount = 0;
}
}
// 次月の日付を埋める
if (rowCount > 0) {
const daysToAdd = 7 - rowCount;
for (let i = 1; i <= daysToAdd; i++) {
calendarHTML += `<td class="next-month">${i}</td>`;
rowCount++;
}
}
calendarHTML += '</table>';
// カレンダー要素にHTMLを挿入
document.querySelector('#calendar').innerHTML = calendarHTML;
}
generateCalendar(2023, 5);
現在時刻の西暦と月を取得したい場合、以下のコードを利用できます。
ここでも、1月を0ではなく1とする為に1を加算しています。
// 現在の年と月の取得
const currentDate = new Date();
const currentYear = currentDate.getFullYear();
const currentMonth = currentDate.getMonth() + 1;
また、動作サンプルでは以下のCSSを適用しています。
#calendar p {
margin: 0 15px;
}
#calendar table {
width: 300px;
table-layout: fixed;
}
#calendar th,
#calendar td {
text-align: center;
}
#calendar .prev-month,
#calendar .next-month {
color: lightgray;
}
ディスカッション
コメント一覧
まだ、コメントがありません