JavaScript | CanvasでrequestAnimationFrameを使った2Dアニメーションサンプル
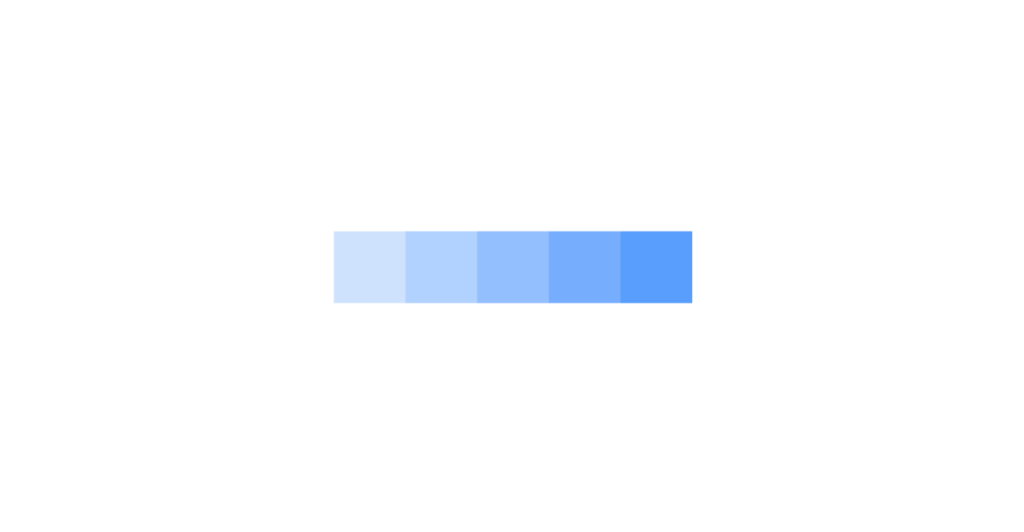
CanvasでrequestAnimationFrame()を使って簡単な2Dアニメーションを実装するシンプルなJavaScriptコードサンプルを紹介しています。
Canvasでアニメーションを実装する
JavaScriptのrequestAnimationFrame()メゾッドを利用してCanvasアニメーションを実装した要素が横移動するだけのシンプルなサンプルコードになります。
See the Pen JavaScript Canvas change size by yochans (@yochans) on CodePen.
HTMLはidを付与したcanvasタグをひとつ用意しています。
<canvas id="canvas"></canvas>
requestAnimationFrame()メゾッドを使ってupdate関数を作成、実行しています。
clearRect()を使ってアップデート時に表示されている描画内容をクリア、新たな位置に描画します。
関数内でrequestAnimationFrame()を呼び出すことでupdate()関数を再実行します。
サンプルでは利用していませんが、ミリ秒単位のアニメーション時間をcallbackで取得可能ですので、経過時間の変数として利用可能です。
Canvasの縦横サイズは指定していませんので、デフォルトサイズの幅300px、高さ150pxとなっています。
Canvasのサイズはwidth、heightで取得、指定、変更する事が可能です。
let canvas = document.querySelector('#canvas');
let context = canvas.getContext('2d');
let x = -50;
function update(callback) {
// 現在の描画をクリアする
context.clearRect(0, 0, canvas.width, canvas.height);
// x(位置)の増加、キャンバスサイズを超えたら戻す
if (x <= canvas.width) {
x = x + 5;
} else {
x = -50;
}
// 描画
context.beginPath();
context.fillStyle = 'dodgerblue';
context.fillRect(x, 50, 50, 50);
// updateを繰り返す
window.requestAnimationFrame(update);
}
// updateの実行
window.requestAnimationFrame(update);
Canvasでアニメーションのアロー関数バージョンです。
let canvas = document.querySelector('#canvas');
let context = canvas.getContext('2d');
let x = -50;
const update = callback => {
// 現在の描画をクリアする
context.clearRect(0, 0, canvas.width, canvas.height);
// x(位置)の増加、キャンバスサイズを超えたら戻す
if (x <= canvas.width) {
x = x + 5;
} else {
x = -50;
}
// 描画
context.beginPath();
context.fillStyle = 'dodgerblue';
context.fillRect(x, 50, 50, 50);
// updateを繰り返す
window.requestAnimationFrame(update);
};
// updateの実行
window.requestAnimationFrame(update);
アニメーションの停止
cancelAnimationFrame()を使う事で、requestAnimationFrame()で実行されているアニメーションを関数毎に停止する事が可能です。
サンプルではupdate()を停止します。
cancelAnimationFrame()はアニメーション関数内、関数外どちらからも利用できます。
window.cancelAnimationFrame(update);